<!DOCTYPE html>
<html lang="zh-en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>canvas练习</title>
<style>
body{
margin: 0px;
}
canvas{
background: #e2dddc;
}
</style>
</head>
<body>
<canvas id="canvas" width="1500px" height="1500px"></canvas>
<script type="text/javaScript">
//固定语法,定义变量,设置绘制环境
var canvas=document.getElementById('canvas');//获取canvas动画的id值,单引号
var context=canvas.getContext('2d');//2d需小写。单引号
//绘制直线
context.lineWidth=5;//设置线的宽度
context.strokeStyle="#156fff";//绘制的样式,颜色,渐变等
context.moveTo(0,0);//设置起点
context.lineTo(200,0);//设置终点
context.stroke();//开始绘制
//可以通过四条直线,但是绘制矩形,会有小缺角
context.beginPath();
context.moveTo(20,20);
context.lineTo(80,20);
context.lineTo(80,80);
context.lineTo(20,80);
// context.lineTo(20,20);//通过绘制四条边,但是在有的浏览器会有小缺角
context.closePath();//可通过closepath闭合图形,与上面最后一条分开
context.stroke();
//矩形绘制通过rect方法
context.beginPath();//重新绘制图形,需要从新定义起点
context.strokeStyle="#696922";//可重新定义样式。。。
context.rect(20,90,50,50);//x,y,w,h的值xy起点,wh矩形的宽高
context.stroke();//开始绘制
//j将rect()和stroke()方法合并
context.beginPath();
context.strokeStyle="#aaaaaa";
context.strokeRect(20,150,50,50);//方法合并绘制矩形
//fillStroke()绘制填充图形
context.beginPath();
context.fillStyle="#EEAEEA";//fillStyle方法类似strokestyle,改变样式
context.fillRect(20,210,50,50);//绘制填充图。默认黑色
/*********************************注意圆弧角度的对比****************************************/
//绘制圆弧arc(x,y,r,开始角度,结束角度,true/false),角度按照弧度Math.PI写,True设置逆时针绘制,false顺时针
context.beginPath();
context.arc(150,50,30,0,Math.PI/3,true);//默认false,顺时针,最后一个参数可以省略
context.stroke();
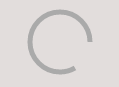
//绘制圆弧arc(x,y,r,开始角度,结束角度,true/false),角度按照弧度Math.PI写,True设置逆时针绘制,false顺时针
context.beginPath();
context.arc(150,120,30,0,Math.PI/3,false);//默认false,顺时针,最后一个参数可以省略
context.stroke();

/*********************************结束***************************************************/
//绘制圆
context.beginPath();
context.fillStyle="#ff3c00"
context.arc(150,190,30,0,Math.PI*2,true);//默认false,顺时针,最后一个参数可以省略
context.fill();//绘制圆填充
context.stroke();//圆形加外框
//不完整圆
context.beginPath();
context.lineWidth=5;
context.arc(150,260,30,0,Math.PI/3,true);//默认false,顺时针,最后一个参数可以省略
context.closePath();//闭合圆外框,
context.fill();//绘制圆填充
context.stroke();
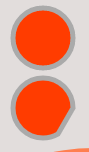
//绘制扇形
context.beginPath();
context.moveTo(250,50);//起点
context.arc(250,50,30,Math.PI*7/6,Math.PI*11/6,false);
context.closePath(); //闭合
context.stroke();
context.fill();
//用fill绘制扇形,两个扇形,通过扇形覆盖完成,但是有细边存在
context.beginPath();
context.fillStyle = "coral";
context.moveTo(600, 200);
context.arc(600, 200, 200, Math.PI * 7 / 6, Math.PI * 11 / 6);
context.closePath();
context.fill();
context.beginPath();
context.fillStyle = "#e2dddc";
context.moveTo(600, 200);
context.arc(600, 200, 100, Math.PI * 7 / 6, Math.PI * 11 / 6);
context.closePath();
context.fill()
//用stroke绘制扇形,直接设置线宽
context.beginPath();
context.strokeStyle = "coral";
context.lineWidth = 100;
context.arc(1000, 200, 150, Math.PI * 11 / 6, Math.PI * 7 / 6, true);
context.stroke();
//汉字添加
context.beginPath();
context.strokeStyle = "coral";
context.lineWidth = 100;
context.arc(200,500,150,Math.PI * 11 / 6, Math.PI * 7 / 6,true);
context.stroke();
context.beginPath();
context.fillStyle="red";
context.strokeStyle="green";
context.font="bold 20px Arial";
context.lineWidth=1;//一定要设置绘制字的宽度,未重新定义宽度,则使用上面定义的宽度,会导致汉字混乱
context.strokeText("纵",180,350);
context.fillText("横",180,390);
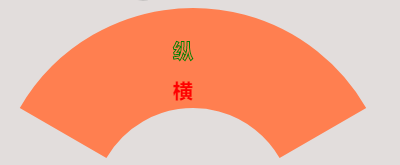
//线性渐变色createLinearCradient()
context.beginPath();
g=context.createLinearGradient(50,650,350,600);//注意大小写.计算线性起点和终点
g.addColorStop(0,"#f00a0a");
g.addColorStop(0.4,"#cbce12");
g.addColorStop(0.8,"#1244ce");
g.addColorStop(1,"#a212ce");
context.strokeStyle = g;
context.lineWidth = 100;
context.arc(200,800,150,Math.PI * 11 / 6, Math.PI * 7 / 6,true);
context.stroke();
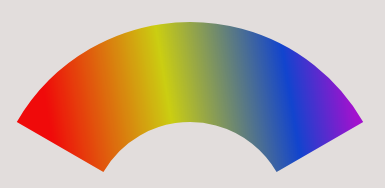
/*****************************************太极图*******************************************/
//canvas绘制太极图,先stroke圆圈,在绘制左右黑白半圆,填充黑白大圆,加入黑白中心小圆
context.beginPath();
context.lineWidth=1;
context.strokeStyle="#000000";
context.arc(600,610,200,0,2*Math.PI);
context.stroke();
context.closePath();
//左边的大黑半圆
context.beginPath();
context.fillStyle="#000000";
context.arc(600,610,200,0.5*Math.PI,1.5*Math.PI);
context.fill();
context.closePath();
//右边的大白半圆
context.beginPath();
context.fillStyle="#ffffff";
context.arc(600,610,200,0.5*Math.PI,1.5*Math.PI,true);
context.fill();
context.closePath();
//上面的左边半圆
context.beginPath();
context.arc(600,510,100,0.5*Math.PI,1.5*Math.PI);
context.fillStyle="white";
context.fill();
context.closePath();
//上面的小黑圆
context.beginPath();
context.arc(600,510,33,0,2*Math.PI);
context.fillStyle="black";
context.fill();
context.closePath();
//下面的右边半黑圆
context.beginPath();
context.arc(600,710,100,Math.PI,(3/2*Math.PI),true);
context.fill();
context.closePath();
//下面的小白圆
context.beginPath();
context.arc(600,710,33,0,2*Math.PI);
context.fillStyle="white";
context.fill();
context.closePath();
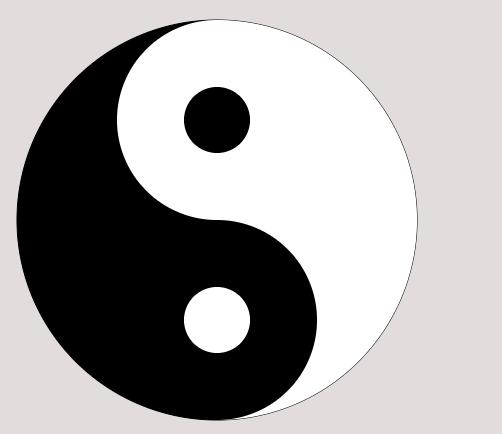
/*****************************************结束*********************************************/
</script>
</body>
</html>