一、Spring Data ElasticSearch简介
1、什么是Spring Data
Spring Data是一个用于简化数据库访问,并支持云服务的开源框架。其主要目标是使得对数据的访问变得方便快捷,并支持map-reduce框架和云计算数据服务。 Spring Data可以极大的简化JPA的写法,可以在几乎不用写实现的情况下,实现对数据的访问和操作。除了CRUD外,还包括如分页、排序等一些常用的功能。
Spring Data的官网:http://projects.spring.io/spring-data/
Spring Data常用的功能模块如下:
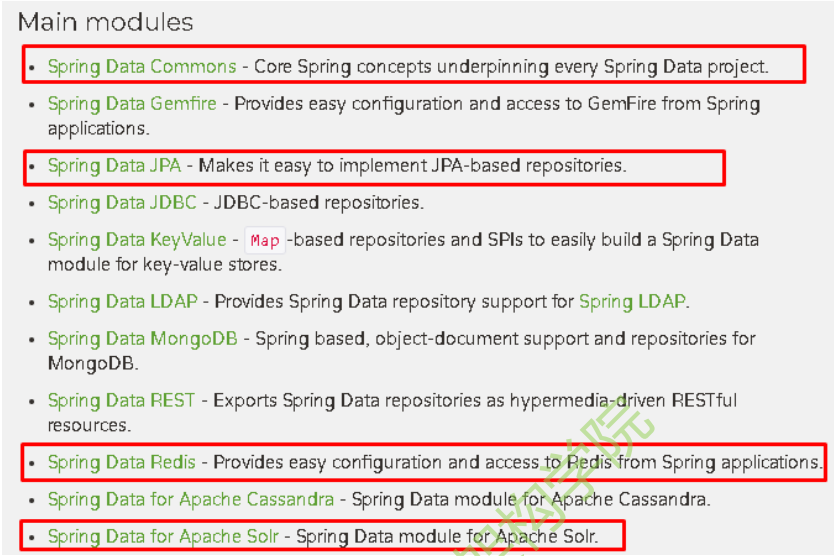
2、什么是Spring Data ElasticSearch
Spring Data ElasticSearch 基于 spring data API 简化 elasticSearch操作,将原始操作elasticSearch的客户端API进行封装 。Spring Data为Elasticsearch项目提供集成搜索引擎。Spring Data Elasticsearch POJO的关键功能区域为中心的模型与Elastichsearch交互文档和轻松地编写一个存储库数据访问层。
官方网站:http://projects.spring.io/spring-data-elasticsearch/
二、Spring Data ElasticSearch环境
1、启动ES集群
2、启动node.js
3、目录展示
4、导入依赖
<dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> <dependency> <groupId>org.elasticsearch</groupId> <artifactId>elasticsearch</artifactId> <version>5.6.8</version> </dependency> <dependency> <groupId>org.elasticsearch.client</groupId> <artifactId>transport</artifactId> <version>5.6.8</version> </dependency> <dependency> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-to-slf4j</artifactId> <version>2.9.1</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>1.7.24</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-simple</artifactId> <version>1.7.24</version> </dependency> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>1.2.12</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> <version>2.8.1</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.8.1</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-annotations</artifactId> <version>2.8.1</version> </dependency> <dependency> <groupId>org.springframework.data</groupId> <artifactId>spring-data-elasticsearch</artifactId> <version>3.0.5.RELEASE</version> <exclusions> <exclusion> <groupId>org.elasticsearch.plugin</groupId> <artifactId>transport‐netty4‐client</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-test</artifactId> <version>5.0.4.RELEASE</version> </dependency> </dependencies>
5、applicationContext.xml配置文件
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:elasticsearch="http://www.springframework.org/schema/data/elasticsearch" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd http://www.springframework.org/schema/data/elasticsearch http://www.springframework.org/schema/data/elasticsearch/spring-elasticsearch.xsd"> <!--扫描Dao包,自动创建实例--> <elasticsearch:repositories base-package="com.zn.dao"/> <!--扫描service包,创建service的实体--> <context:component-scan base-package="com.zn.service"/> <!--配置elasticsearch的连接--> <elasticsearch:transport-client id="client" cluster-nodes="127.0.0.1:9300,127.0.0.1:9301,127.0.0.1:9302" cluster-name="my-elasticsearch"/> <!--ElasticSearch模板对象--> <bean id="elasticsearchTemplate" class="org.springframework.data.elasticsearch.core.ElasticsearchTemplate"> <constructor-arg name="client" ref="client"></constructor-arg> </bean> </beans>
6、Article实体
package com.zn.entity; import org.springframework.data.annotation.Id; import org.springframework.data.elasticsearch.annotations.Document; import org.springframework.data.elasticsearch.annotations.Field; import org.springframework.data.elasticsearch.annotations.FieldType; //@Document 文档对象 (索引信息,文档类型) @Document(indexName = "es_test03",type = "article") public class Article { @Id //@Id文档主键 唯一标识 //@Field 每个文档的字段配置(类型、是否分词、是否存储、分词器 ) @Field(type = FieldType.Integer,store = true,index = false) private Integer id; @Field(type = FieldType.text,index = true,store = true,analyzer = "ik_max_word") private String title; @Field(type = FieldType.text,index = true,store = true,analyzer = "ik_max_word") private String content; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getContent() { return content; } public void setContent(String content) { this.content = content; } @Override public String toString() { return "Article{" + "id=" + id + ", title='" + title + '\'' + ", content='" + content + '\'' + '}'; } }
三、创建索引库
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; public interface ArticleService { }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; /*创建索引库*/ @Test public void creatIndex(){ //创建空的索引库 template.createIndex(Article.class); } }
5、效果展示
四、创建索引库并指定mappings
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; public interface ArticleService { }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; /*创建索引库并添加mapping映射*/ @Test public void creatIndexAndMapping(){ template.createIndex(Article.class); template.putMapping(Article.class); } }
5、效果展示
五、创建文档
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; public interface ArticleService { //创建文档 public void save(Article article); }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; @Override public void save(Article article) { dao.save(article); } }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; /*创建文档*/ @Test public void saveDocument(){ Article article=new Article(); article.setId(1); article.setTitle("测试SpringData ElasticSearch"); article.setContent("Spring Data ElasticSearch基于spring data API简化elasticSearch操作," + "将原始操作elasticSearch的客户端API进行封装"); service.save(article); } }
5、效果展示
六、删除文档--根据id
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; public interface ArticleService {
//根据文档id删除 public void deleteById(Integer id); }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; @Override public void deleteById(Integer id) { dao.deleteById(id); } }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; /*根据id删除文档*/ @Test public void deleteDocumentById(){ //根据文档ID删除 service.deleteById(1); } }
5、效果展示
七、删除全部文档
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; public interface ArticleService { //删除全部文档 public void deleteAll(); }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; @Override public void deleteAll() { dao.deleteAll(); } }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; /*删除全部文档*/ @Test public void deleteAllDocument(){ //全部删除 service.deleteAll(); } }
5、效果展示
八、修改文档
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; public interface ArticleService { //修改文档 public void save(Article article); }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; @Override public void save(Article article) { dao.save(article); } }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; /*修改文档,先删除再修改,调用的还是save方法*/ @Test public void updateDocument(){ Article article=new Article(); article.setId(1); article.setTitle("1[修改]测试SpringData ElasticSearch"); article.setContent("1[修改]Spring Data ElasticSearch基于spring data API简化elasticSearch操作," + "将原始操作elasticSearch的客户端API进行封装"); service.save(article); } }
5、效果展示
九、根据ID查询文档
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; import java.util.Optional; public interface ArticleService { //根据ID查询文档 public Optional<Article> findById(Integer id); }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import java.util.Optional; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; @Override public Optional<Article> findById(Integer id) { return dao.findById(id); } }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; import java.util.Optional; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; /*根据ID查询文档*/ @Test public void findById(){ Optional<Article> optional=service.findById(2); Article article = optional.get(); System.out.println(article); } }
5、效果展示
十、查询全部文档
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable; import java.util.Optional; public interface ArticleService { //查询全部文档 public Iterable<Article> findAll(); }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable; import org.springframework.stereotype.Service; import java.util.Optional; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; @Override public Iterable<Article> findAll() { return dao.findAll(); } }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; import java.util.Optional; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; /*查询全部文档*/ @Test public void findAllDocument(){ Iterable<Article> all = service.findAll(); all.forEach(item-> System.out.println(item)); } }
5、效果展示
十一、分页查询全部文档
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable; import java.util.Optional; public interface ArticleService { //分页查询全部文档
public Page<Article> findAll(Pageable pageable); }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable; import org.springframework.stereotype.Service; import java.util.Optional; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; @Override public Page<Article> findAll(Pageable pageable) { return dao.findAll(pageable); } }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Page; import org.springframework.data.domain.PageRequest; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; import java.util.Optional; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; /*分页查询全部文档*/ @Test public void findAllDocumentByPage(){ Page<Article> all = service.findAll(PageRequest.of(0, 5)); for (Article list:all.getContent()) { System.out.println(list); } } }
5、效果展示
十二、自定义规则查询--Title域
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.domain.Pageable; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; import java.util.List; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { //自定义规则 //根据Title域中的关键词查找 public List<Article> findByTitle(String title); }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable; import java.util.List; import java.util.Optional; public interface ArticleService { //自定义规则 //根据Title域中的关键词查找 public List<Article> findByTitle(String title); }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable; import org.springframework.stereotype.Service; import java.util.List; import java.util.Optional; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; @Override public List<Article> findByTitle(String title) { return dao.findByTitle(title); } }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Page; import org.springframework.data.domain.PageRequest; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; import java.util.List; import java.util.Optional; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; //自定义规则 /*根据Title域中的关键词查找*/ @Test public void findByTitle(){ List<Article> lists= service.findByTitle("修改");
lists.stream().forEach(item-> System.out.println(item));
} }
5、效果展示
十三、分页自定义规则查询--Title域
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.domain.Pageable; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; import java.util.List; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { //自定义规则 //分页根据Title域中的关键词查找 public List<Article> findByTitle(String title, Pageable pageable); }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable; import java.util.List; import java.util.Optional; public interface ArticleService { //自定义规则 //分页根据Title域中的关键词查找 public List<Article> findByTitle(String title, Pageable pageable); }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable; import org.springframework.stereotype.Service; import java.util.List; import java.util.Optional; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; @Override public List<Article> findByTitle(String title, Pageable pageable) { return dao.findByTitle(title,pageable); } }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Page; import org.springframework.data.domain.PageRequest; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; import java.util.List; import java.util.Optional; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; //自定义规则 /*分页根据Title域中的关键词查找*/ @Test public void findByTitleAndPage(){ List<Article> title = service.findByTitle("测试", PageRequest.of(0, 5)); title.stream().forEach(item-> System.out.println(item)); } }
5、效果展示
十四、根据一段内容查询--NativeSearchQuery(Elasticsearch原生查询)
1、ArticleDao
package com.zn.dao; import com.zn.entity.Article; import org.springframework.data.domain.Pageable; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import org.springframework.stereotype.Repository; import java.util.List; @Repository public interface ArticleDao extends ElasticsearchRepository<Article,Integer> { }
2、ArticleService
package com.zn.service; import com.zn.entity.Article; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable; import java.util.List; import java.util.Optional; public interface ArticleService { }
3、ArticleServiceImpl
package com.zn.service.impl; import com.zn.dao.ArticleDao; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Page; import org.springframework.data.domain.Pageable; import org.springframework.stereotype.Service; import java.util.List; import java.util.Optional; @Service public class ArticleServiceImpl implements ArticleService { @Autowired private ArticleDao dao; }
4、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.elasticsearch.index.query.QueryBuilders; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Page; import org.springframework.data.domain.PageRequest; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.data.elasticsearch.core.query.NativeSearchQuery; import org.springframework.data.elasticsearch.core.query.NativeSearchQueryBuilder; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; import java.util.List; import java.util.Optional; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; /*根据一段内容查询--NativeSearchQuery*/ @Test public void findByNativeSearchQuery(){ //创建NativeSearchQueryBuilder对象 NativeSearchQueryBuilder nativeSearchQueryBuilder=new NativeSearchQueryBuilder(); //指定查询规则 (先分词再搜索) NativeSearchQuery build = nativeSearchQueryBuilder.withQuery(QueryBuilders.queryStringQuery("搜索测试").defaultField("title")).build(); //使用ElasticsearchTemplate执行查询
List<Article> articles = template.queryForList(build, Article.class); articles.stream().forEach(item-> System.out.println(item)); } }
5、效果展示
十五、分页根据一段内容查询--NativeSearchQuery(Elasticsearch原生查询)
1、SpringDataESTest
package com.zn; import com.zn.entity.Article; import com.zn.service.ArticleService; import org.elasticsearch.client.transport.TransportClient; import org.elasticsearch.index.query.QueryBuilders; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Page; import org.springframework.data.domain.PageRequest; import org.springframework.data.elasticsearch.core.ElasticsearchTemplate; import org.springframework.data.elasticsearch.core.query.NativeSearchQuery; import org.springframework.data.elasticsearch.core.query.NativeSearchQueryBuilder; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; import java.util.List; import java.util.Optional; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = "classpath:applicationContext.xml") public class SpringDataESTest { @Autowired private ArticleService service; @Autowired private ElasticsearchTemplate template; /*分页根据一段内容查询--NativeSearchQuery*/ @Test public void findByNativeSearchQueryAndPage(){ //创建NativeSearchQueryBuilder对象 NativeSearchQueryBuilder nativeSearchQueryBuilder=new NativeSearchQueryBuilder(); //指定查询规则 (先分词再搜索) NativeSearchQuery build = nativeSearchQueryBuilder.withQuery(QueryBuilders.queryStringQuery("搜索测试").defaultField("title")) .withPageable(PageRequest.of(0,5)) .build(); //使用ElasticsearchTemplate执行查询 List<Article> articles = template.queryForList(build, Article.class); articles.stream().forEach(item-> System.out.println(item)); } }
2、效果展示