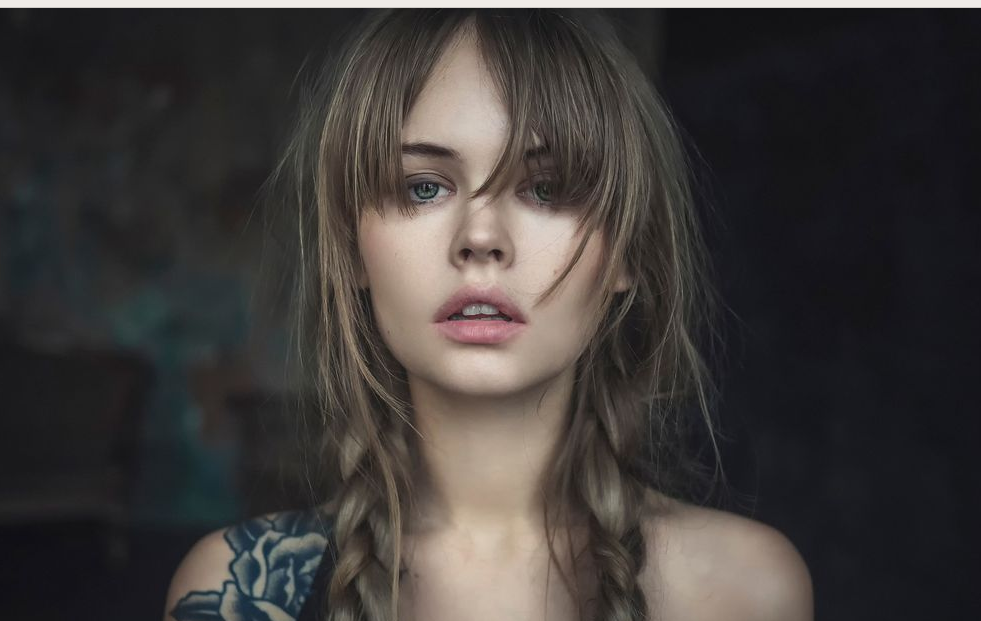
Kmeans
# coding: utf-8
import cv2
import numpy as np
img = cv2.imread("/home/hichens/Datasets/pic/11.jpg")
if len(img.shape) == 3:
data = img.reshape(-1, 3)
else:
data = img.reshape(-1, 1)
data = np.float32(data)
#定义中心 (type,max_iter,epsilon)
criteria = (cv2.TERM_CRITERIA_EPS +
cv2.TERM_CRITERIA_MAX_ITER, 10, 1.0)
#设置标签
flags = cv2.KMEANS_RANDOM_CENTERS
#K-Means聚类 聚集成4类
Ncenter = 2
compactness, labels, centers = cv2.kmeans(data, Ncenter, None, criteria, 10, flags)
#生成最终图像
res = centers[labels.flatten()]
dst = res.reshape(img.shape)
print(dst.shape)
dst = np.array(dst, dtype=np.uint8)
dst = cv2.cvtColor(dst, cv2.COLOR_BGR2RGB)
while True:
cv2.imshow("img", img)
cv2.imshow("dst", dst)
if cv2.waitKey(1) == 27:
break
cv2.destroyAllWindows()
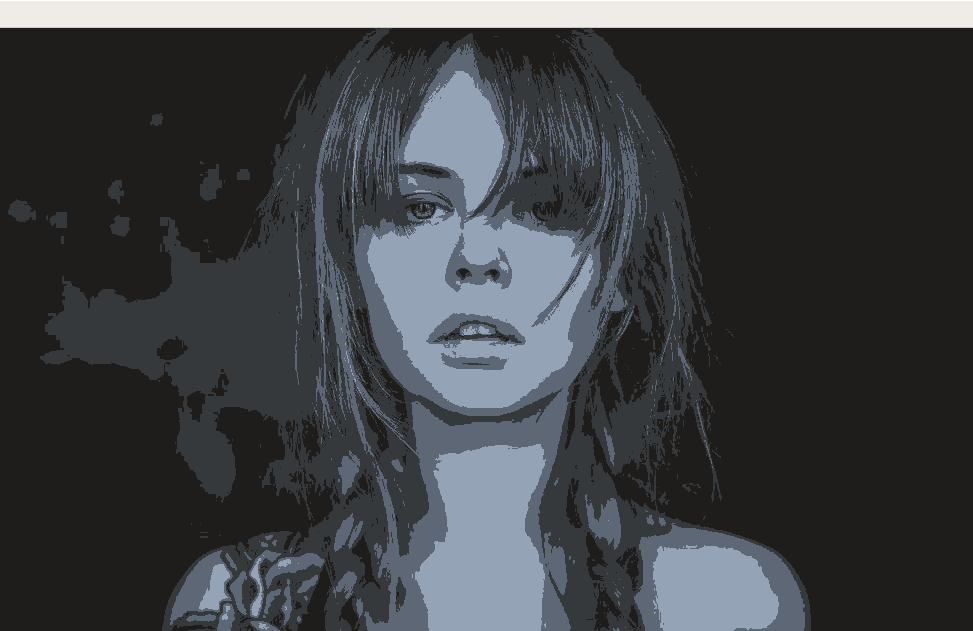
FCM
import cv2
import skfuzzy as fuzz
import numpy as np
img = cv2.imread('/home/hichens/Datasets/pic/11.jpg')
if len(img.shape) == 3:
data = img.reshape(-1, 3)
else:
data = img.reshape(-1, 1)
data = np.float32(data)
Ncenter = 4
cntr, u, _, _, _, _, fpc = fuzz.cluster.cmeans(
data.T, Ncenter, 2, error=0.05, maxiter=100, init=None)
labels = np.argmax(u, axis=0)
res = cntr[labels.flatten()]
dst = res.reshape(img.shape)
print(dst.shape)
print(fpc)
dst = np.array(dst, dtype=np.uint8)
dst = cv2.cvtColor(dst, cv2.COLOR_BGR2RGB)
while True:
cv2.imshow("img", img)
cv2.imshow("dst", dst)
if cv2.waitKey(1) == 27:
break
cv2.destroyAllWindows()
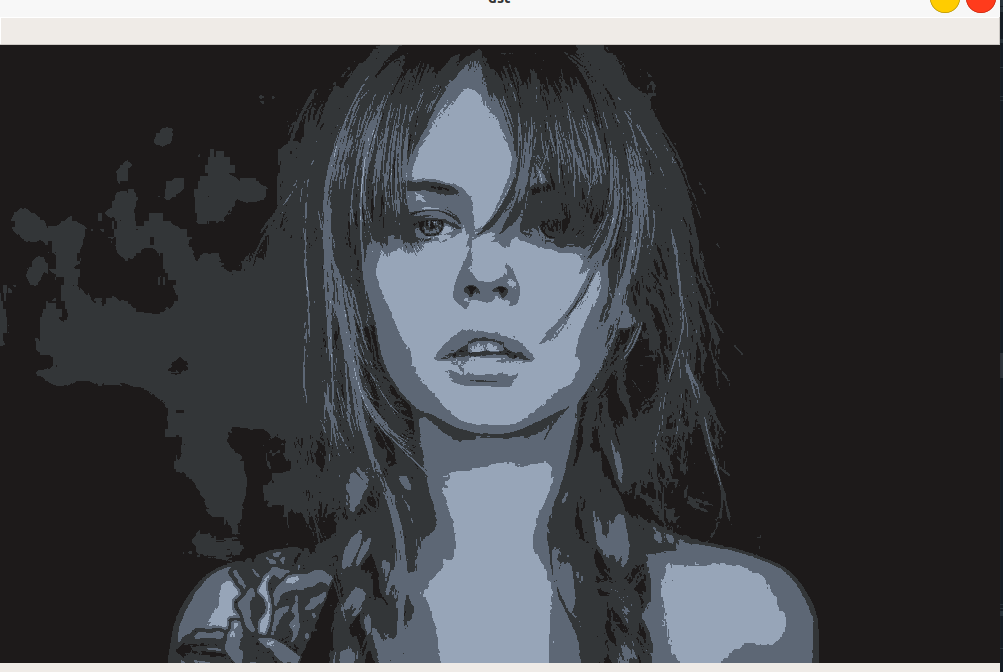