准备灰度图 grayTest.png,放置于Assets下StreamingAssets文件夹中。
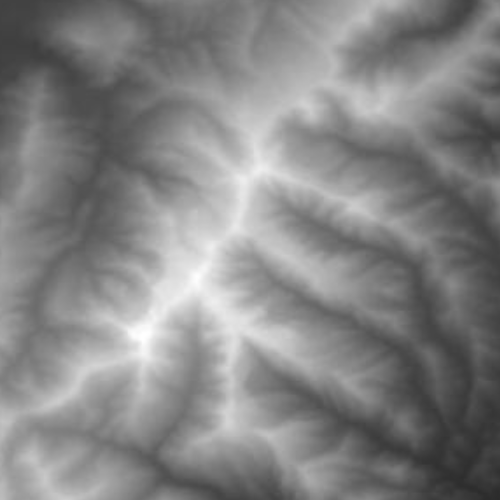
在场景中添加RawImage用于显示最后的等值线图。
生成等值线的过程,使用Marching squares中Isolines方式。
首先将顶点数据与阈值比较,对顶点进行标记。根据四个顶点的标记情况,连接各个线段的中点,组成等值线。
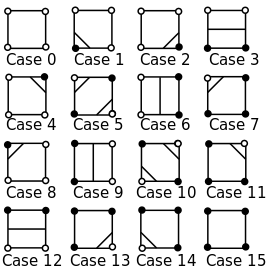
测试中在texture2d对线段中点进行涂色以显示。
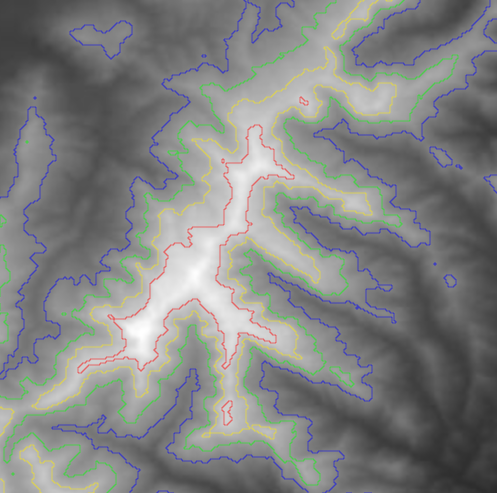
脚本如下:
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; //生成等值线图 public class IsoLinesCreate : MonoBehaviour { private Texture2D importImage;//输入灰度图 private Texture grayImage;//加载灰度图 private Texture2D outputImage;//输出等值线图 private bool bCreate=false; [Tooltip("用作显示输出的等高线图")] public RawImage showImage; private int tGrayWidth = 0, tGrayHeight = 0;//灰度图的宽和高 void Start () { StartCoroutine(loadImage("grayTest.png", (t) => grayImage = t)); } void Update () { if (grayImage!=null) { if (bCreate==false) { importImage = (Texture2D)grayImage; outputImage = (Texture2D)grayImage; tGrayWidth = grayImage.width; tGrayHeight = grayImage.height; showImage.rectTransform.sizeDelta = new Vector2(tGrayWidth,tGrayHeight); //测试不同阈值不同颜色等值线绘制 trackIsoline(0.5f,Color.blue,2); trackIsoline(0.6f, Color.green, 2); trackIsoline(0.7f, Color.yellow, 2); trackIsoline(0.8f, Color.red, 2); showImage.texture = outputImage; bCreate = true; } } } //加载图片 IEnumerator loadImage(string imagePath, System.Action<Texture> action) { WWW www = new WWW("file://" + Application.streamingAssetsPath + "/" + imagePath); yield return www; if (www.error == null) { action(www.texture); } } /// <summary> /// 等值线追踪 /// </summary> /// <param name="threshold">灰度阈值</param> /// <param name="colorP">颜色</param> /// <param name="step">步长 为偶数</param> private void trackIsoline(float threshold,Color colorP,int step) { for (int i = 0; i < tGrayWidth-step; i+=step) { for (int j = 0; j < tGrayHeight-step; j+=step) { //标记顶点状态 int tempCount = 0;//0000 if (importImage.GetPixel(i,j).grayscale>threshold) { tempCount += 1;//0001 } if (importImage.GetPixel(i+step, j).grayscale > threshold) { tempCount += 8;//1000 } if (importImage.GetPixel(i+step, j+step).grayscale > threshold) { tempCount += 4;//0100 } if (importImage.GetPixel(i, j+step).grayscale > threshold) { tempCount += 2;//0010 } int t = step / 2; //根据顶点标记情况处理中点连线(此处对中点进行上色) switch (tempCount) { case 0: case 15: break; case 1: case 14: outputImage.SetPixel(i+t,j,colorP); outputImage.SetPixel(i,j+t,colorP); break; case 2: case 13: outputImage.SetPixel(i , j+t, colorP); outputImage.SetPixel(i+t, j + 2*t, colorP); break; case 3: case 12: outputImage.SetPixel(i+t, j, colorP); outputImage.SetPixel(i + t, j + 2 * t, colorP); break; case 4: case 11: outputImage.SetPixel(i +2* t, j+t, colorP); outputImage.SetPixel(i + t, j + 2 * t, colorP); break; case 5: outputImage.SetPixel(i + t, j, colorP); outputImage.SetPixel(i + 2*t, j + t, colorP); outputImage.SetPixel(i , j + t, colorP); outputImage.SetPixel(i + t, j + 2 * t, colorP); break; case 6: case 9: outputImage.SetPixel(i, j + t, colorP); outputImage.SetPixel(i +2* t, j + t, colorP); break; case 7: case 8: outputImage.SetPixel(i+t, j, colorP); outputImage.SetPixel(i + 2 * t, j + t, colorP); break; case 10: outputImage.SetPixel(i + t, j, colorP); outputImage.SetPixel(i, j + t, colorP); outputImage.SetPixel(i + 2*t, j+t, colorP); outputImage.SetPixel(i + t, j + 2*t, colorP); break; } } } outputImage.Apply(); } }
本文链接 https://www.cnblogs.com/gucheng/p/10107696.html